Event Tracking API
Note
Applicable Hawksearch Versions: All
Applicable Integration types: Hawksearch V4.0 API and API - front end integration
Overview
The event tracking API is used to support critical features in HawkSearch, for example:
- Reports
- Recommendations
- AI Multiplier
Tracking Process Overview
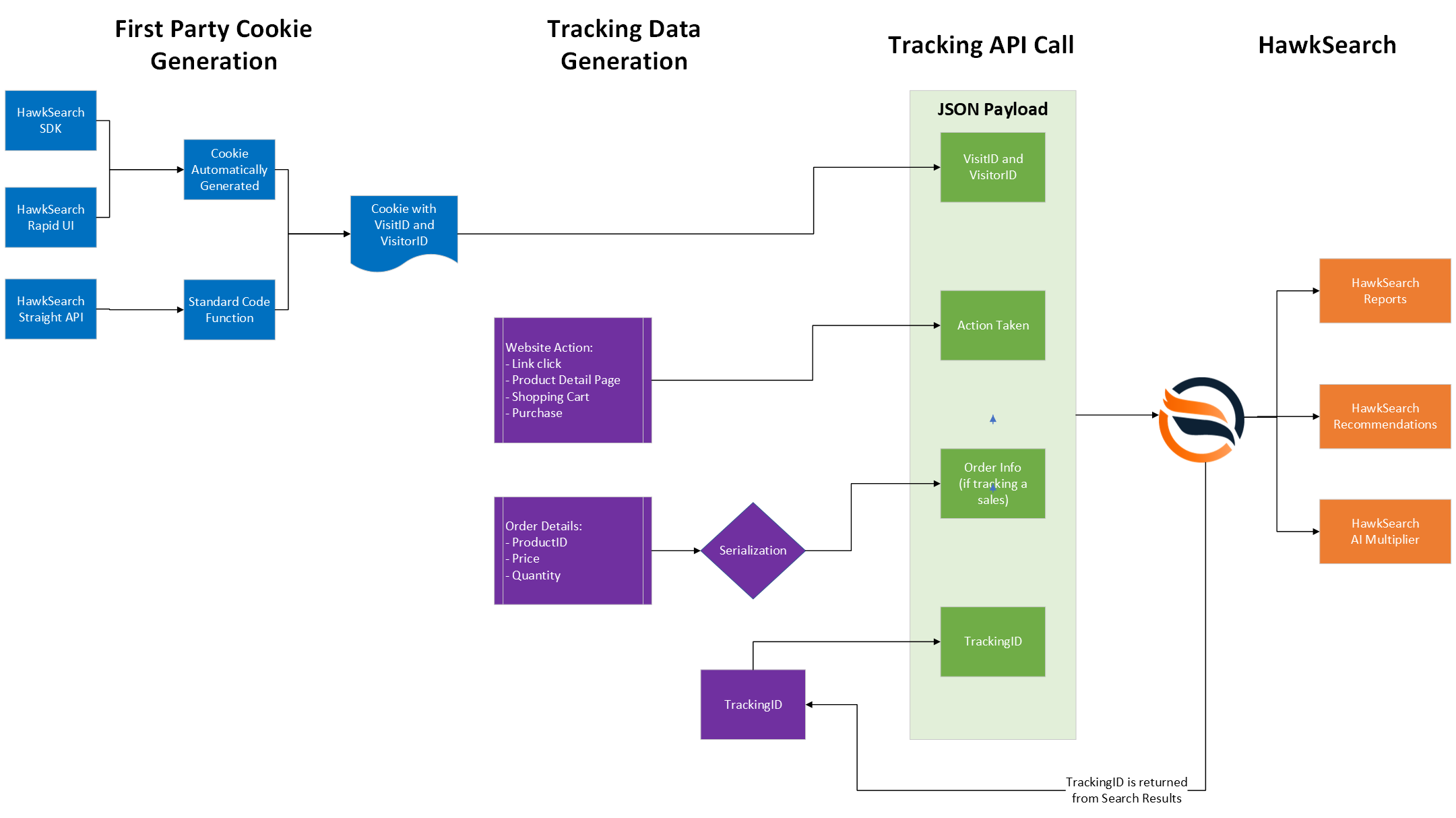
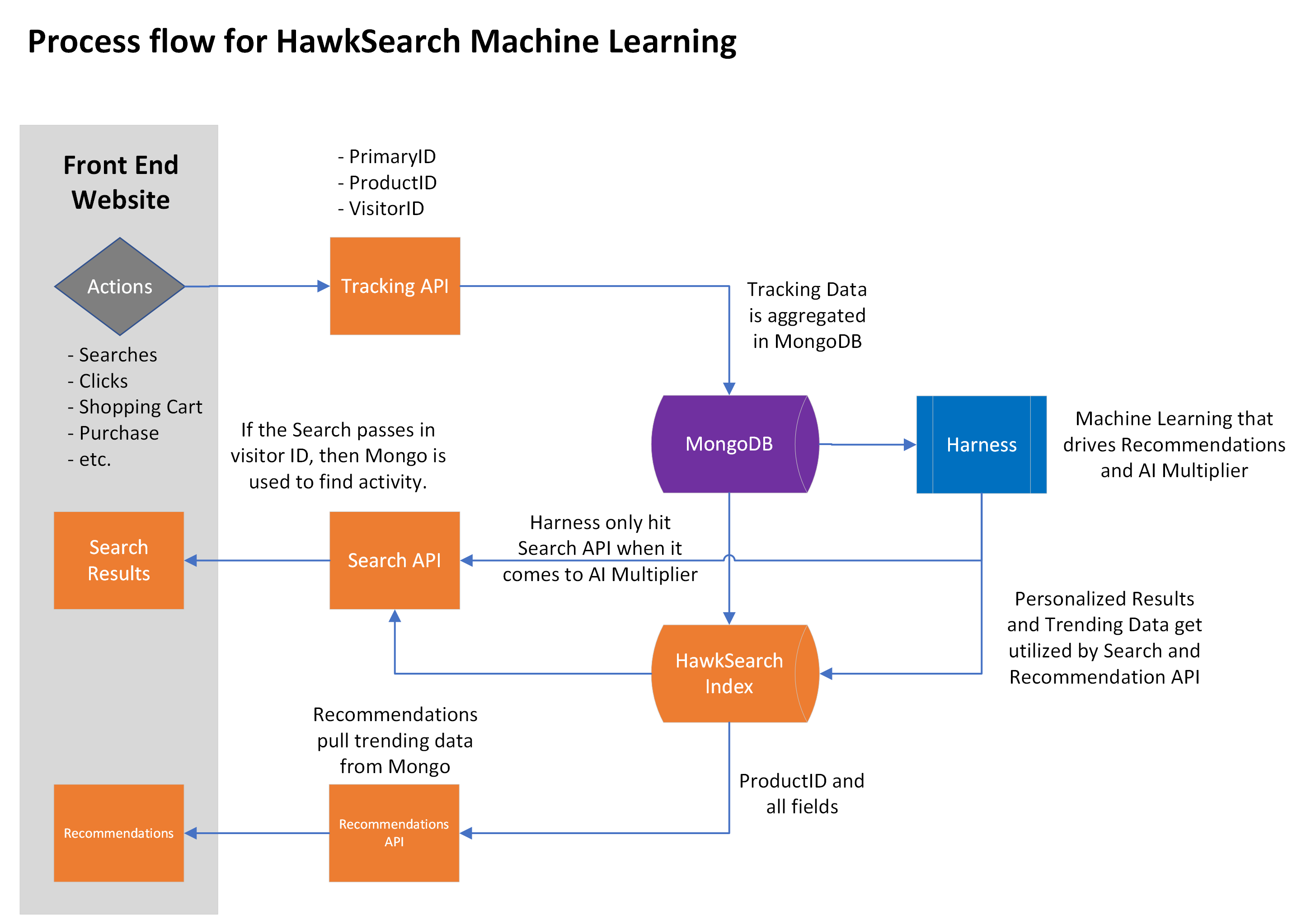
Domains
HawkSearch has three environments available: Development, Test and a load-balanced Production. When using the search API methods in this document, the following domains can be used to access each environment after your engine has been set-up in that environment.
Before You Begin
Please ensure that your client-specific endpoints are configured before implementing any API integrations. Please refer to this article to learn more about client-specific endpoints and reach out to our CSD team for any questions.
Development
client-specific URL: https://enginename.dev.tracking-na.hawksearch.com
Configurations for an engine in this location are maintained in the HawkSearch Workbench at dev.hawksearch.net.
Test
client-specific URL: https://enginename.test.dashboard-na.hawksearch.com
Configurations for an engine in this location are maintained in the HawkSearch Workbench at test.hawksearch.net.
Production
client-specific URL: https://enginename.tracking-na.hawksearch.com
Configurations for an engine in this location are maintained in the HawkSearch Workbench at dashboard-na.hawksearch.com.
Tracking API Components
Important
TrackingId is unique to every search request and required in tracking requests.
TrackingId
Since Search and Tracking are separate requests, and based on HTTP which is stateless inherently, there is a need to establish a relationship with the search request and a corresponding tracking request. This can be achieved by an identifier unique to the pair of search and tracking requests.
For every search request made to HawkSearch's Search API, along with the search results, merchandising section, etc., HawkSearch also gives back a unique identifier in the response. This identifier should be sent back through tracking requests which corresponds to the search made.
{
...
"TrackingId" : "0d6a30c0-5a6e-4995-8802-7d7096b7fb86",
...
}
This TrackingId needs to be used in sending the tracking requests back to HawkSearch's tracking service so that HawkSearch can establish the connectivity between the search and tracking requests.
Please note to include this dynamic id obtained from HawkSearch's response, into the tracking requests.
Using a TrackingId from an old search response or a random guid may not be recorded in the reports on HawkSearch workbench.
This is different from VisitId, VisitorId and the TrackingGuid attributes.
How to track requests
The steps to correctly track a Search event is as below, please refer to the specific event details in the remainder of this article for more examples.
Identifiers and their scope
All of these IDs need to be generated and sent to HawkSearch, since API integration typically does not require/use HawkSearch provided Javascript code:
VisitorId - this is the Guid to be set once per user. We recommend this to be stored in a browser cookie so that this same id is used when the user closes and reopens the browser and visits your website. Typically, once the vlaue of this cookie is set, it does not change. This is set to not expire or expire after a long term - like a year. HawkSearch uses this to ensure tracking events correspond to a given user and help recommend user-specific products through recommendation widgets.
Note - that VisitorId does not map to a registered user on your website so the same registered user opening your website in different browsers or in incognito mode will have different visitor id's. To ensure that HawkSearch system knows all these are the same user, please use our identify
API in conjunction with the Ids explained later (Tracking Member Logins) in this article.
VisitId - this is the Guid set for every visit or “_user _session” of a logged in/guest user. This corresponds to the user opening the website and performing search, click, add to cart and any other operation. This gives our reports generator the information about the user’s activity on his/her certain visit to the website. This is scoped only until the browser is open. If the browser is closed and a fresh new browser window is opened, then this value needs to be set newly.
QueryId - is a unique id per “_keyword _session” - a session limited only to the specific search term. If the user searches for a keyword and continues to make facet selections, change the sort order and paging etc, all will fall under the same QueryId. QueryId changes when search term changes.
Notes:
- Scope of User Id (identify api) > Scope of VisitorId > Scope of VisitId > Scope of QueryId
- Note: TypeId is 1 for “Regular Searches” i.e., when a new search is performed. It is 2 for “Refined Search” - facet filtering, sorting, paging selection changes for the same keyword until the user searches for something else.
Identifier | Value Range | Generated By | Typical Persistence Location | Scope | Expiry (reset when) | Usage (sent to Hawk during) |
---|---|---|---|---|---|---|
Query Id | Valid GUID | Website | Page Viewstate/Viewbag/temporary | Keyword | User searches for different keyword (search term) | Tracking API requests |
Visit Id | Valid GUID | Website | Browser Cookie | Browser specific tab/window | Opening a new browser tab/window | Search and Tracking API requests |
Visitor Id | Valid GUID | Website | Browser Cookie | Browser - all tabs and windows | Browser cache is cleared | Search and Tracking API requests |
UserId/MemberId/AccountId with identify API | Alphanumeric - individual website specific | Website | Website’s backend database (Userid) and HawkSearch’s database (Mapping between website’s User id and HawkSearch’s visitor id & visit id) | Website level | Never | One time mapping request through Tracking API |
TrackingId | Valid GUID | HawkSearch | Page Viewstate/Viewbag/temporary | Keyword | User searches for different keyword (search term) | Sent by Hawksearch in search response, to be sent back by website to Hawksearch for the corresponding tracking API request (see Tracking Id section above) |
TypeId | 1 or 2 | Website | Page Viewstate/Viewbag/temporary | Keyword | Reset to 1 when - User searches for different keyword (search term) Change to 2 upon - faceting/pagination/sorting changes by the user for the same search result set (keyword hasnt changed yet and user is clickign on facets/sorting/etc.) | Tracking API requests |
Field Specific Search Explained: When to Use TypeId 1 vs TypeId 2
Field Specific Search allows users to search directly within a specific data field, rather than performing a broad keyword search. This is particularly useful when the user already knows what type of field they want to filter by, such as a brand, category, or part number (but not through facet selections). Think of it like using Google's Advanced Search, where you can narrow your search to certain parts of a page, exclude words, or limit results by site or region.
For example, instead of just typing rat terrier, you can tell the search engine without searching "all these words":
- “Only show results where this exact phrase appears” ("rat terrier")
- Or “Exclude pages that mention Jack Russell” (-"Jack Russell")
- Or “Only search within a specific website” (site:wikipedia.org)
These types of searches are considered field specific because you are telling the engine to look for information in a specific place or format.
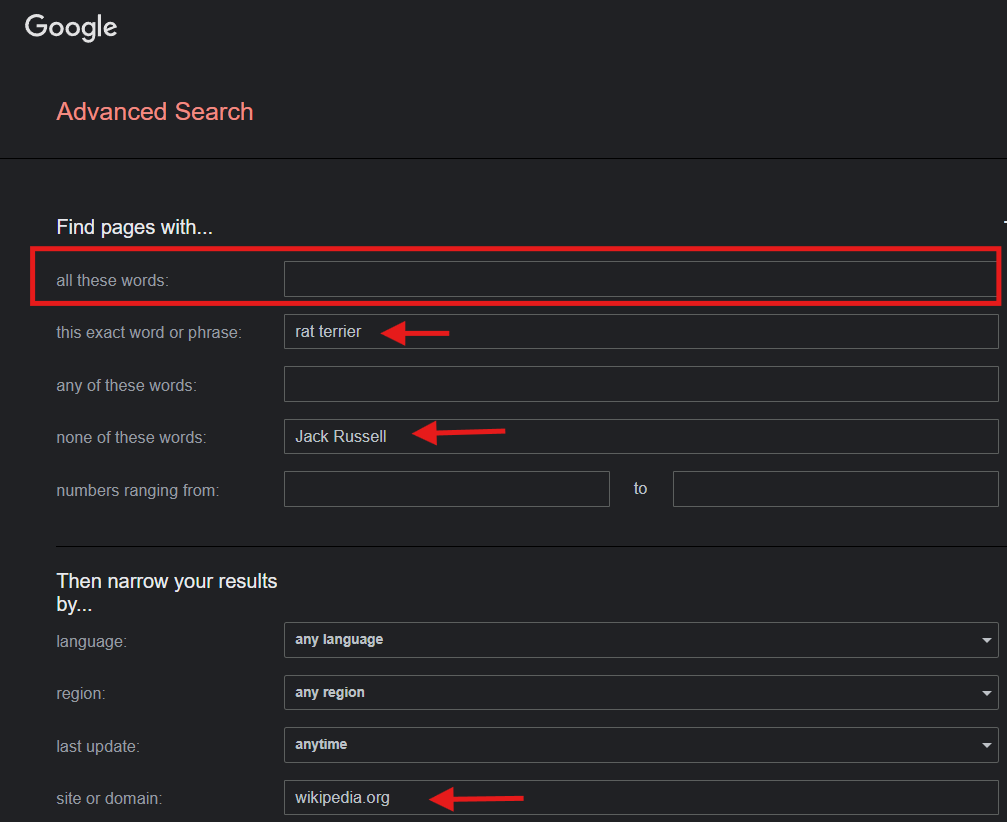
This leads to confusion: “If there’s no keyword, is it even a search and should the TypeId be 1 or 2?”
The answer is yes — because the user is still expressing intent and triggering a new result set. That’s why it’s considered a valid Search Event, specifically a TypeId = 1. Even without a keyword, a field-specific query is a starting point of user intent and therefore should be logged as TypeId 1.
✅ When Is It TypeId 1 vs TypeId 2?
To simplify:
Situation Description TypeId User starts a search (keyword or field-specific) No filters/sorting/pagination 1 User clicks on facets or changes sorting or selects different page in pagination Refining the current search 2 Tip to remember:
TypeId 1 = “A new search starts”
TypeId 2 = “I'm adjusting the results of my current search”
Additional Note
If a user refreshes the page with the same keyword or field-specific query, this is still considered a new search (not a refinement), and should be tracked as TypeId 1. A page reload represents a new search session from the system’s perspective, even if the input hasn’t changed.
Code samples for Cookie and GUIDs for TrackingId, VisitorId and VisitId
If you are leveraging a HawkSearch SDK or Rapid UI, then those solutions include the creation of the first party cookie and correct VisitorId and includes functions to generate the correct VisitId. If you are building a solution using the HawkSearch API only, you can generate this information as you like. We have included code samples if interested.
VisitorId, VisitId and TrackingId helper
/*
Local and Session Storage based Tracking API helper
- this helper class will assist in generating and storing GUID values for Visit and Visitor Ids
- this helper class will not automatically set the Query Id returned from the Search API
- Query Id is a GUID provided in the Search API response whenever someone performs a search. This GUID should be persistent until another search is performed
*/
class HawkSearchTracking {
#hawksearch_query_id_name;
#hawksearch_visit_id_name;
#hawksearch_visitor_id_name;
constructor(visit_id_name, visitor_id_name) {
this.#hawksearch_query_id_name = 'HawkSearchQueryId'; // Query Id Storage Name
this.#hawksearch_visit_id_name = visit_id_name ?? 'HawkSearchVisitId'; // Visit Id Storage Name
this.#hawksearch_visitor_id_name = visitor_id_name ?? 'HawkSearchVisitorId'; // Visitor Id Storage Name
}
// Clear Visit Id - Call this function after an order has been placed
clearHawksearchVisitId() {
sessionStorage.removeItem(this.#hawksearch_visit_id_name);
};
// Retrieve HawkSearch Query Id
getHawkSearchQueryId() {
return localStorage.getItem(this.#hawksearch_query_id_name);
}
// Retrieve HawkSearch Visit Id
getHawksearchVisitId() {
let visit_id = sessionStorage.getItem(this.#hawksearch_visit_id_name);
if (!visit_id) {
visit_id = this.#createHawksearchUUID();
}
sessionStorage.setItem(this.#hawksearch_visit_id_name, visit_id);
return visit_id;
};
// Retrieve HawkSearch Visitor Id
getHawksearchVisitorId () {
let visitor_id = localStorage.getItem(this.#hawksearch_visitor_id_name);
if (!visitor_id) {
visitor_id = this.#createHawksearchUUID();
}
localStorage.setItem(this.#hawksearch_visitor_id_name, visitor_id);
return visitor_id;
};
// Set HawkSearch Query Id
setHawkSearchQueryId(value) {
localStorage.setItem(this.#hawksearch_query_id_name, value);
}
// Helper functions
#createHawksearchUUID() {
// Public Domain/MIT - RFC4122 v4 compliant
let d = new Date().getTime();
let d2 = ((typeof performance !== 'undefined') && performance.now && (performance.now()*1000)) || 0;
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
let r = Math.random() * 16;
if(d > 0){
r = (d + r)%16 | 0;
d = Math.floor(d/16);
} else {
r = (d2 + r)%16 | 0;
d2 = Math.floor(d2/16);
}
return (c === 'x' ? r : (r & 0x3 | 0x8)).toString(16);
});
}
}
Cookie helper
/*
Cookie based HawkSearch Tracking API helper
- this helper class will assist in generating and storing GUID values for Visit and Visitor Ids
- this helper class will not automatically set the Query Id returned from the Search API
- Query Id is a GUID provided in the Search API response whenever someone performs a search. This GUID should be persistent until another search is performed
*/
class HawkSearchTracking {
#hawksearch_query_id_name;
#hawksearch_visit_id_name;
#hawksearch_visitor_id_name;
constructor(visit_id_name, visitor_id_name) {
this.#hawksearch_query_id_name = 'HawkSearchQueryId'; // Query Id Storage Name
this.#hawksearch_visit_id_name = visit_id_name ?? 'HawkSearchVisitId'; // Visit Id Storage Name
this.#hawksearch_visitor_id_name = visitor_id_name ?? 'HawkSearchVisitorId'; // Visitor Id Storage Name
}
// Clear Visit Id - Call this function after an order has been placed
clearHawksearchVisitId() {
this.#setHawksearchCookie(this.#hawksearch_visit_id_name, "", 'Thu, 01 Jan 1970 00:00:01 GMT');
};
// Retrieve HawkSearch Query Id
getHawkSearchQueryId() {
return this.#getHawksearchCookie(this.#hawksearch_query_id_name);
}
// Retrieve HawkSearch Visit Id
getHawksearchVisitId() {
let visit_id = this.#getHawksearchCookie(this.#hawksearch_visit_id_name);
if (!visit_id) {
visit_id = this.#createHawksearchUUID();
}
this.#setHawksearchCookie(this.#hawksearch_visit_id_name, visit_id, this.#getHawksearchVisitExpiry());
return visit_id;
};
// Retrieve HawkSearch Visitor Id
getHawksearchVisitorId() {
let visitor_id = this.#getHawksearchCookie(this.#hawksearch_visitor_id_name);
if (!visitor_id) {
visitor_id = this.#createHawksearchUUID();
}
this.#setHawksearchCookie(this.#hawksearch_visitor_id_name, visitor_id, this.#getHawksearchVisitorExpiry());
return visitor_id;
};
// Set HawkSearch Query Id
setHawkSearchQueryId(value) {
this.#setHawksearchCookie(this.#hawksearch_query_id_name, value);
}
// Helper functions
#createHawksearchUUID() {
// Public Domain/MIT - RFC4122 v4 compliant
let d = new Date().getTime();
let d2 = ((typeof performance !== 'undefined') && performance.now && (performance.now()*1000)) || 0;
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
let r = Math.random() * 16;
if(d > 0){
r = (d + r)%16 | 0;
d = Math.floor(d/16);
} else {
r = (d2 + r)%16 | 0;
d2 = Math.floor(d2/16);
}
return (c === 'x' ? r : (r & 0x3 | 0x8)).toString(16);
});
}
#getHawksearchCookie(name) {
let nameEQ = name + "=";
let ca = document.cookie.split(';');
for (let i = 0; i < ca.length; i++) {
let c = ca[i];
while (c.charAt(0) == ' ') c = c.substring(1, c.length);
if (c.indexOf(nameEQ) == 0) return c.substring(nameEQ.length, c.length);
}
return null;
};
#getHawksearchVisitExpiry() {
var d = new Date();
d.setTime(d.getTime() + (4 * 60 * 60 * 1000)); // 4 hours
return d.toGMTString();
};
#getHawksearchVisitorExpiry() {
var d = new Date();
d.setTime(d.getTime() + (360 * 24 * 60 * 60 * 1000)); // 1 year
return d.toGMTString();
};
#setHawksearchCookie(name, value, expiry) {
let expires;
if (expiry) {
expires = "; expires=" + expiry;
} else {
expires = "";
}
document.cookie = name + "=" + value + expires + "; path=/";
};
}
Tracking API Methods
POST
The POST method is used to pass tracking events to your recommendation engine. Hawk Search allows for tracking many different types of events (listed below) using only one generic method common for all types of events.
Response Code
Structure
Response Code | Object | Data type | Description |
---|---|---|---|
200 | null | null | null |
400 | Message | String | A detailed explanation on why the event failed to insert, Example below:{ "Message": "Invalid ClientGuid"} |
Example
Go to Tracking Event Types section to see examples.
{
"ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b",
"VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5",
"VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43",
"EventType":"PageLoad",
"EventData": "",
...
}
Tracking Event Types
HawkSearch API allows you to collect information about different types of events in a system. Depending on the type, the API expects related data about the occurring event.
EventType property represents following values (both EventType Value and EventyType Code can be used to define field values)
EventType Values
EventType Code | EventType Code | EventType Value |
---|---|---|
Page Load Event | PageLoad | 1 |
Search Event | Search | 2 |
Click Event | Click | 3 |
Add To Cart Event | Add2Cart | 4 |
Rate Event | Rate | 5 |
Sale Event | Sale | 6 |
Banner Click Event | BannerClick | 7 |
Banner Impression Event | BannerImpression | 8 |
Tracking Member Login Event | Identify | 9 |
Recommendation Click Event | RecommendationClick | 10 |
Autocomplete Click Event | AutoCompleteClick | 11 |
Add To Cart Multiple Event | Add2CartMultiple | 14 |
Banner Impression Bulk Event | BannerImpressionBulk | 16 |
Autocomplete Trending Category Click Event | AutoCompleteTrendingCategoryClick | 17 |
Autocomplete Trending Items Click Event | AutoCompleteTrendingItemsClick | 18 |
This section describes different types of events and their representing model.
Page Load Event
Events representation for tracking specific pages customers are loading.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
PageTypeId | number | required | Number between 1 and 5 indicating the page type: 1 - Item detail page (i.e. PDP) - please pass UniqueId for this type of page 2 - Landing Page 3 - Shopping Cart Page 4 - Order Confirmation Page 5 - All other Page Types |
Qs | string | optional | Query string passed within page url |
RequestPath | string | required | The URL of requested page |
ViewportHeight | number | optional | The height of the device viewport. |
ViewportWidth | number | optional | The width of the device viewport. |
UniqueId | string | required when PageTypeId=1 | Represents the unique identifier of a product. |
CustomDictionary | Array of objects | optional | Can be used to send information used for evaluating Visitor Targets. |
Examples
-
Event Data Object
{ "PageTypeId":"1", "Qs":"varA=1&varB=2", "RequestPath":"/details/sku123", "UniqueId":"sku123", "ViewportHeight":1080, "ViewportWidth":1920 }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"PageLoad", "EventData":... // Serialized and Base64 Encoded PageLoad Event Data Object }
-
Example of CustomDictionary
-
Event Data Object
{ "PageTypeId":"1", "Qs":"varA=1&varB=2", "RequestPath":"/details/sku123", "UniqueId":"sku123", "ViewportHeight":1080, "ViewportWidth":1920 }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"PageLoad", "EventData":... // Serialized and Base64 Encoded PageLoad Event Data Object "CustomDictionary": {“custom“:”search”} }
-
Search Event
To track search events in web application you should call tracking API using this type of event.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
TrackingId | string | required | The ‘TrackingId’ object that is passed in this function is returned in the HawkSearch response |
TypeId | int | required | Should be 1 when an initial search is performed, should be 2 when a refinement (i.e. selecting facets, changing pages, changes sort by, etc) is performed |
QueryId | guid | required | Query ID is a guid generated whenever someone performs a search. This GUID is persistent until another search is performed |
RemoteAddr | string | optional | The IP address of the client that is being tracked |
Qs | string | optional | Query string passed within page url |
RequestPath | string | optional | The URL of requested page |
ViewportHeight | number | optional | The height of the device viewport. |
ViewportWidth | number | optional | The width of the device viewport. |
CustomDictionary | object | optional | To send information used for evaluating Visitor Targets. |
> custom | string | optional | The name of the Visitor Target to be passed. |
Example
-
Event Data Object
{ "Qs":"", "TypeId":"1", "QueryId":"26a0a9f9-f22d-45c2-bee7-35832a2171e3", "RemoteAddr":"127.0.0.1", "RequestPath":"/", "TrackingId":"6d017a40-54ba-4508-9272-fc14f4616661", "ViewportHeight":1080, "ViewportWidth":1920 }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"Search", "EventData":..... // Serialized and Base64 Encoded Search Event Data Object "CustomDictionary": { "custom": "VisitorTarget1" } }
Click Event
To track click events in web application you should call tracking API using this type of event.
This click event is applicable for user clicks made on the search results on search page or HawkSearch landing page.
For user clicks on Banners, Recommendation widget items and Autocomplete sections, please refer to appropriate events.
Event Data Model
Parameters
Note
All of the properties that name starts with Element* should pass corresponding information about clicked element
Property Name | Data Type | Required | Description |
---|---|---|---|
TrackingId | guid | required | This is the TrackingId that was passed back in the search response. |
UniqueId | string | required | Unique identifier of item that was clicked |
ElementNo | number | required | The position of the clicked search result within the results. Formula : ElementNo = item position on current page + (max items per page (current page number - 1)) E.g. if the item is the 5th result in 2nd page of 12 items, then the value is 5 + 12 (2-1) = 17. |
Mlt | boolean | optional | Mlt stands for "More Like This" and it determines if more like this element was clicked. |
MouseX | number | optional | Horizontal Mouse Position |
MouseY | number | optional | Vertical Mouse Position |
Qs | string | optional | Query string passed within page url |
RequestPath | string | optional | The URL of requested page |
ScrollX | number | optional | Horizontal scroll position |
ScrollY | number | optional | Vertical scroll position |
Url | string | optional | Target URL that clicked element points to |
ViewportHeight | number | optional | The height of the device viewport. |
ViewportWidth | number | optional | The width of the device viewport. |
Example
-
Event Data Object
{ "Mlt":false, "TrackingId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "Url":"/details/sku123.html", "UniqueId":"sku123", "ElementNo": 17 }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"Click", "EventData":..... // Serialized and Base64 Encoded Click Event Data Object }
Add To Cart Event
This type of tracking should be populated by events of adding items to the cart.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
Currency | string | required | Actual currency in ISO format |
Price | number | required | Represents a single item price |
Quantity | number | required | The number of items being added to the cart |
UniqueId | string | required | Represents the unique identifier of a product. This should correspond to the value of the field set up as the primary key in the fields section of HawkSearch dashboard |
Examples
-
Event Data Object
{ "UniqueId":"123456789", "Price":15.97, "Quantity":1, "Currency":"USD" }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"Add2Cart", "EventData": ... // Serialized and Base64 Encoded Add To Cart Event Data Object }
Add To Cart Multiple Event
In some unique instances, the user is able to add many items to the cart with one click.
This method will allow you to call API tracking with single event with multiple items.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
ItemsList | array of objects | required | Is an array of objects that stores information about items. To see object properties check Item Info Model below. |
Item Info Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
Price | number | required | Price of an individual item |
Quantity | number | required | The number of items |
UniqueId | string | required | Represents the unique identifier of a product. |
Currency | string | required | Actual currency in ISO format |
Examples
-
Event Data Object
{ "ItemsList":[ { "uniqueId":"123456789", "price":15.97, "quantity":1, "currency":"USD" }, { "uniqueId":"54234", "price":10.97, "quantity":2, "currency":"USD" } ] }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"Add2CartMultiple", "EventData":..... // Serialized and Base64 Encoded Add To Cart Multiple Event Data Object }
🛒 AddToCart vs AddToCartMultiple
Overview: Two tracking events are available to capture cart additions:
- Add To Cart: Tracks a single item added to cart.
- Add To Cart Multiple: Allows submitting multiple items in one call.
Behavior:
- There is no functional difference between AddToCart and AddToCartMultiple if only one item is passed in the request.
- Both will result in the same event tracking outcome.
Recommendation: Use AddToCartMultiple when batching multiple items into one event. Use AddToCart for individual additions. However, using either for a single item is valid and interchangeable.
Sale Event
In order to track sales events, call the tracking API using the following event eg. on your confirmation page.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
Currency | string | optional | The actual currency in ISO format |
SubTotal | number | optional | The order the sub total amount |
Tax | number | optional | The tax value |
Total | number | optional | The actual order total amount |
OrderNo | string | required | The alphanumeric value representing the order number |
ItemList | array of objects | required | Is an array of objects that stores information about items. To see object properties check Sale Item Info Model below. |
Sale Item Info Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
ItemPrice | number | required | Price of an individual item |
Quantity | number | required | The number of sold items |
UniqueId | string | required | Represents the unique identifier of a product. |
Example
-
Event Data Object
{ "SubTotal":100.33, "Tax":23.3, "Total":123.3, "OrderNo":"A0000378", "Currency":"USD", "ItemList":[ { "uniqueId":"123456789", "ItemPrice":15.97, "quantity":1 } ] }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"Sale", "EventData":..... // Serialized and Base64 Encoded Sale Event Data Object }
Rate Event
HawkSearch can provide personalized recommendations to users who rated items on the website. In order to send ‘rate’ event, please call the Tracking API using following event type.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
UniqueId | string | required | The unique identifier of an item. In many cases the uniqueId is different than SKU. |
Value | number | required | Represents a user’s rating for an item. The decimal value must be between 1 and 5. |
Examples
-
Event Data Object
{ "UniqueId":"Item_1232", "Value":3.00 }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"Rate", "EventData":..... // Serialized and Base64 Encoded Sale RateData Object }
Banner Impression Event
To track banner impressions in web application you should call tracking API using this type of event, each time users display banner.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
TrackingId | string | required | The ‘TrackingId’ object that is passed in this function is returned in the HawkSearch response |
BannerId | int | required | The identifier of corresponding banner |
CampaignId | int | optional | The identifier of corresponding campaign |
Examples
- Event Data Object
{ "BannerId":1250, "CampaignId":12, "TrackingId":"6d017a40-54ba-4508-9272-fc14f4616661", }
- Complete Request Body
{
"ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b",
"VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5",
"VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43",
"EventType":"BannerImpression",
"EventData":..... // Serialized and Base64 Encoded Banner Impression Event Data Object
}
Banner Click Event
To track banner clicks in web application you should call tracking API using this type of event.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
TrackingId | string | required | The ‘TrackingId’ object that is passed in this function is returned in the HawkSearch response |
BannerId | int | required | The identifier of corresponding banner |
CampaignId | int | optional | The identifier of corresponding campaign |
Examples
-
Event Data Object
{ "BannerId":1250, "CampaignId":12, "TrackingId":"6d017a40-54ba-4508-9272-fc14f4616661", }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"BannerClick", "EventData":..... // Serialized and Base64 Encoded Banner Click Event Data Object }
Banner Impression Bulk Event
To track a list of banner impressions in web application you should call tracking API using this type of event, each time users display a single or multiple banners.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
Banners | Array of Objects | required | The object will contain all below attributes. See below example. |
TrackingId | string | required | The ‘TrackingId’ object that is passed in this function is returned in the HawkSearch response |
Banner Info Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
> > BannerId | int | required | The identifier of corresponding banner |
> > CampaignId | CampaignId | required | The identifier of corresponding campaign |
Examples
-
Event Data Object
{ "TrackingId":"6d017a40-54ba-4508-9272-fc14f4616661", "Banners": [ { "BannerId":1250, "CampaignId":12 }, { "BannerId":1257, "CampaignId":14 } ] }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"BannerImpressionBulk", "EventData":..... // Serialized and Base64 Encoded Banner Impression Event Data Object }
Recommendation Click Event
To track recommendation widget click action you should call tracking API using following type of event, each time user clicks on recommendation widget.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
ItemIndex | int | optional | The number of the clicked recommendation item on the displayed widget. |
RequestId | guid | required | The ‘RequestId’ object that is passed in this function is returned in the recommendation widget response |
UniqueId | string | required | Represents the unique identifier of a product. This should correspond to the value of the field set up as the primary key in the fields section of HawkSearch dashboard. |
WidgetGuid | guid | required | The unique identifier of the recommendation widget that displayed clicked item. This should correspond to the widget GUID generated for each widget individually in HawkSearch dashboard. |
Examples
-
Event Data Object
{ "ItemIndex": 1, "RequestId":'', "UniqueId":"6d017a40-54ba-4508-9272-fc14f4616661", "WidgetGuid":'', }
-
Complete Request Body
{ "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"RecommendationClick", "EventData":..... // Serialized and Base64 Encoded Banner Impression Event Data Object }
Autocomplete Click Event
Use this event to track user clicks on different type of autocomplete results eg. Products, Categories, Content, Popular Searches.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
Keyword | string | required | Selected keyword used as a autocomplete query. |
Name | string | required | Text value associated with the autocomplete item clicked |
SuggestType | int | optional | Number between 1 and 4 indicating the autocomplete results type: 1 - Popular Searches 2 - Top Categories 3 - Top Product Matches 4 - Top Content Matches |
Url | string | required | The target URL of clicked autocomplete item |
Examples
-
Event Data Object
{ "Keyword": "jacket", "Name":"Man » Jacket", "SuggestType":2, "Url":'<https://dev.hawksearch.net/elasticdemo?department_nest=Jackets_6'>, }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"AutocompleteClick", "EventData":..... // Serialized and Base64 Encoded Autocomplete Event Data Object }
Autocomplete Trending Items Click Event
Use this event to track user clicks on autocomplete trending items results. When autocomplete returns products and IsRecommended = ture then Autocomplete Trending Items click event should be used.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
ItemId | string | required | ItemId associated with the autocomplete item clicked |
Url | string | required | The target URL of clicked autocomplete item |
Examples
-
Event Data Object
{ "ItemId": "Item_146566", "Url":'<https://preview-dev.hawksearch.net/elasticdemo/details?itemid=Item_146566>' }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"AutoCompleteTrendingItemsClick", "EventData":..... // Serialized and Base64 Encoded Autocomplete Event Data Object }
Autocomplete Trending Category Click Event
Use this event to track user clicks on autocomplete trending category results. When autocomplete returns category and IsRecommended = ture then Autocomplete Trending Category click event should be used.
Event Data Model
Parameters
Property Name | Data Type | Required | Description |
---|---|---|---|
Field | string | required | Field value returned by autocomplete |
Value | string | required | Value associated with the autocomplete category clicked |
Url | string | required | The target URL of clicked autocomplete category |
Examples
-
Event Data Object
{ "Field": "jacket", "Value":"Camp & Hike » Tents & Shelters", "Url":'<https://preview-dev.hawksearch.net/elasticdemo?department_nest=Tents--Shelters&prv=1>" }
-
Complete Request Body
{ "ClientGuid":"dfb51ea2ea1a42e4a53d4e67f7f6847b", "VisitId":"2F87556F-AA2F-438E-A52C-AFF4B7E10EB5", "VisitorId":"7e3efd4c-ca6b-46fe-b576-1a00ce8d1d43", "EventType":"AutoCompleteTrendingCategoryClick", "EventData":..... // Serialized and Base64 Encoded Autocomplete Event Data Object }
Tracking Member Logins (if applicable)
Bind anonymous users to registered users on your web site
By default, each individual user is tracked via “visit_id” and “visitor_id” cookies. These cookies are used to track the activity of the user. If you have a member sign-up on your site, you can attach the member login to the user’s activity. In order to provide a relationship between the logged-in member and the user’s cookie values, please make an API call to the following method:
https://tracking-dev.hawksearch.net/api/identify
In order to use the API you will need to pass the API key in the header of your request to authenticate your request. To lookup the API key for your account please look in the HawkSearch workbench under Admin >> Account Info.
Sample request
PUT /api/identify HTTP/1.1
Host: tracking-dev.hawksearch.net
X-HawkSearch-ApiKey: 535bd002-1fc5-41a1-b264-1cd29dbd0e65
Content-Type: application/json; charset=utf-8
Cache-Control: no-cache
{"userId":311043,"visitId":"b014cb04-6c73-4e84-8cba-a6858bb1f785","visitorId":"e98f3943"}
userId: the Unique Identifier of the logged in user that is stored in your database (i.e. UserAccountId or MemberId, etc.)
vistitId: this is taken from the visit_id cookie
vistitorId: this is take from the visitor_id cookie
This request should be a server-side request.
Common Action Flows
Search Result Click Flow
A user visits an e-commerce site selling electronics. They load the homepage (#1), search for "wireless headphones" (#2), click on a pair of headphones (#3) from the search results, view the product details page (#4), add the headphones to their cart (#5), and complete the purchase (#6).
- Page load event (other page type)
- Search event
- Click event (item clicked in search results)
- Page load event (product detail page)
- Add to cart event
- Sale event
Autocomplete Click Flow
A user visits an online bookstore homepage (#1). As they start typing "sci-fi nov" in the search bar, they see "science fiction novel" in the autocomplete suggestions. They click on this suggestion (#2), are taken to a page to view its details (#3), add it to their cart (#4), and complete the purchase (#5).
- Page load event (other page type)
- Autocomplete click event (item selected from autocomplete suggestion)
- Page load event (product detail page)
- Add to cart event
- Sale event
Recommendation Click Flow
A user is shopping on a fashion website and viewing a landing page (#1). On this page, they see a "Frequently Bought Together" recommendation widget (#2) showing accessories. They click on a recommended necklace (#3), got redirect to its product detail page (#4), decide to add it to their cart (#5), and then complete the purchase the item (#6).
- Page load event (landing page)
- Recommendation impression event (this is tracked on server side, no need to send request)
- Recommendation click event (item clicked in recommendation widget)
- Page load event (product detail page)
- Add to cart event
- Sale event
✨ Recommendation Impression Tracking
Overview: Recommendation Impression events track when recommendation is rendered to the user. This event is handled server-side and is not required to be sent manually.
Behavior:
- These events are automatically triggered and tracked whenever a recommendation widget is requested from the server and shown on the page.
- No manual API call is needed to track these impressions.
Note: Although visible in the Tracking Event Preview (located in Admin), this event is managed by the server logic and not part of the client-facing tracking script implementation.
FAQs
Where do I find the ClientGuid (Tracking Key)?
When logged into the HawkSearch Workbench, click on the account icon found on the right side of the top bar, next to the logout icon. When your account page loads, look at the bottom where the Tracking Key/Guid is displayed.
How to Test Tracking?
Presence of events on Tracking Preview page
All tracking events can be viewed and tested by logging into the HawkSearch workbench and navigating to
Admin >> Tracking Events Preview
<https://dev.hawksearch.net/admin/trackingPreview.aspx>
Perform a search from the preview page and refresh the tracking preview page
It must display the search event corresponding to the keyword search you just made.
Similarly test for presence of the Event Click and other events on the tracking preview page.
Please ensure all the tracking events are coming through. Some important events are:
- Event Page Load
- Search
- Event Click
- Add To Cart
- Event Sale
Combinations of tracking events confirm to every report. So if a report is not displaying data or showing incorrect data, please check to ensure your website is sending the tracking events to your HawkSearch engine.
Note
The event Request Tracking is logged by HawkSearch internally for summarization purposes.
Presence of UniqueId
TIP: clicking the eyeball icon will pop-up a window with details of the request.
To test every event, you can use the search bar on the Tracking Preview to filter the event type.
To test every event, you can use the search bar on the Tracking Preview to filter the event type.
The most important thing to check is that the UniqueId is being passed in the correct manner. This MUST match what is set to the primary key in the HawkSearch workbench.
Note that, in the tracking requests, the name of the attribute remains “UniqueId” however its value will need to be that associated with the primary key. So:
- If “SKU” is set as primary key, then HawkSearch expects that field in the tracking requests, example:
if your item’s SKU is ABC-1
{
...
"UniqueId" : "ABC-1",
...
}
- If “Id” is set as primary key, then HawkSearch expects that field in the tracking requests, example:
if your item’s Id is 10001
{
...
"UniqueId" : "10001",
...
}
The primary key field is defined under the Field Configuration settings.
Important
If you have a parent-child setup in your HawkSearch index, ensure that you send the correct id according to your setup.
Examples:
If you have all child items' attributes roll up into your parent so that you display only the parent item, then the uniqueid you need to send in tracking should be the parent id even if the item displayed is the child.
If you have all child items displayed in the search results, then send the child item’s id as the uniqueid to the tracking API.
For the latest version of Variant Logic with HawkSearch v4.0 API, sending the parent’s unique id helps generate correct reporting data and recommendations.
Note
UniqueId may not be applicable to all events - for example, a search event may not need to have a uinique id associated with it however for a click event, HawkSearch must know which item was clicked upon.
HawkSearch Event - V1 Click Tracking
HawkSearch has three environments available: Development dev.hawksearch.net), Test – test.hawksearch.net and Production dashboard-na.hawksearch.com. When performing integration, each engine can be accessed by using these domains. For each of the three environments the value for 'url_value' would be different. Please confirm the URL values with the HawkSearch team during implementation.
One of the first steps to populate click tracking report, would be loading the JavaScript file provided by HawkSearch and to initialize the Hawk URLs on the listing page
<script type="text/javascript">
//<![CDATA[
(function (HawkSearch, undefined) {
HawkSearch.BaseUrl = url_value;
HawkSearch.TrackingUrl = url_value;
if ("https:" == document.location.protocol) {
HawkSearch.BaseUrl = HawkSearch.BaseUrl.replace("http://", "https://");
HawkSearch.TrackingUrl = HawkSearch.TrackingUrl.replace("http://", "https://");
}
}(window.HawkSearch = window.HawkSearch || {}));
var hawkJSScriptDoc = document.createElement("script");
hawkJSScriptDoc.async = true;
hawkJSScriptDoc.src = HawkSearch.TrackingUrl + '/includes/hawksearch.js?v1.01';
var hawkJSTag = document.getElementsByTagName('script')[0];
hawkJSTag.parentNode.insertBefore(hawkJSScriptDoc, hawkJSTag);
var hawkCSSScriptDoc = document.createElement("link");
hawkCSSScriptDoc.setAttribute("rel", "stylesheet");
hawkCSSScriptDoc.setAttribute("type", "text/css");
hawkCSSScriptDoc.setAttribute("href", HawkSearch.TrackingUrl + '/includes/hawksearch.css');
document.head.appendChild(hawkCSSScriptDoc);
//]]>
</script>
If the listing page is completely formatted by HawkSearch then the following on click event would be added to the anchor elements for each of the items.
If the site is not using HTML formatted by HawkSearch, then you will require to add the following on click event on each of the item links for products on the listing page.
<a onclick="return HawkSearch.link(event,'61816065-4fa5-4bf2-9604-f76e664f9334',1,’123456789',0);">
Item Name
</a>
The parameters used in the above code snippet are
Parameter 1: passes the event to the function. For click tracking, this value is usually event.
Parameter 2: This is the TrackingId that is passed back in the search response from HawkSearch
61816065-4fa5-4bf2-9604-f76e664f9334
Parameter 3: this is the index of the item in the search results.
Parameter 4: this is the uniqueId of the item.(this may not necessarily be the product Id)
Parameter 5: is always 0 (zero)
Once the above mentioned tracking code has been added, you can test the click tracking event by
- Searching for a term
- Once items have been listed from the search open up the developer tools for the browser
- This can be done either by right clicking on the page and selecting inspect or clicking F12
Chrome
Firefox
- Once the developer tools has been opened up, click on the network tab to view the network requests/response
- Click on any of the products item links on which we have added the click tracking code mentioned previously in the document. On successful tracking the network tabs would show a request to link.aspx with a response status of 302 as shown below.
- If you are not able to see the link.aspx request under network tab make sure that the product listing doesn’t have multiple item links and if they do have multiple item links then the click tracking code is added to each of the links . For example it may happen that a page will have same item link url added on the page for the item image and the item name, then it is required that the click tracking code is added on both item image and item name.
- If the network tab does display the link.aspx request and the item is not redirecting to the product page please make sure there are no error messages on the browser console
Cookie Expiration Table
Cookie Name | Cookie Type | Cookie Expiration | Usage/ Purpose |
---|---|---|---|
hawk_query_id | Session | Session | Query id for tracking |
hawk_visitor_id | Persistent | 1 year | Visitor id for tracking |
hawk_visit_id | Persistent | 24 hours | Visit id for tracking |
recentsearches | Persistent | 12 hours | Recent searches if feature enabled |
Updated 2 months ago